SliceHighlightCurrentProxyModel
Qt Base Class: QIdentityProxyModel
Signature: QIdentityProxyModel(self, parent: Optional[PySide6.QtCore.QObject] = None) -> None
Base classes
Name | Children | Inherits |
---|---|---|
SliceIdentityProxyModel prettyqt.itemmodels.proxies.sliceidentityproxymodel |
⋔ Inheritance diagram
graph TD
1473290744272["itemmodels.SliceHighlightCurrentProxyModel"]
1473290716944["itemmodels.SliceIdentityProxyModel"]
1473299892128["core.IdentityProxyModel"]
1473299903840["core.AbstractProxyModelMixin"]
1473299890176["core.AbstractItemModelMixin"]
1473299815024["core.ObjectMixin"]
140713234304496["builtins.object"]
1473289064768["QtCore.QIdentityProxyModel"]
1473289061840["QtCore.QAbstractProxyModel"]
1473289050128["QtCore.QAbstractItemModel"]
1473288842240["QtCore.QObject"]
1473291690208["Shiboken.Object"]
1473290716944 --> 1473290744272
1473299892128 --> 1473290716944
1473299903840 --> 1473299892128
1473299890176 --> 1473299903840
1473299815024 --> 1473299890176
140713234304496 --> 1473299815024
1473289064768 --> 1473299892128
1473289061840 --> 1473289064768
1473289050128 --> 1473289061840
1473288842240 --> 1473289050128
1473291690208 --> 1473288842240
140713234304496 --> 1473291690208
🛈 DocStrings
Bases: SliceIdentityProxyModel
Proxy model which highlights all cells with same data as current index.
Highlights all cells with same content in given role as currently focused cell with a user-specified color.
Possible modes are:
all
: Highlight all cells with same value.column
: Highlight all cells with same value and same column as current.row
: Highlight all cells with same value and same row as current.
dct = dict(
a=["a", "b", "a", "b"],
b=["a", "b", "a", "b"],
c=["a", "b", "a", "b"],
d=["b", "a", "b", "a"],
e=["a", "b", "a", "a"],
)
model = gui.StandardItemModel.from_dict(dct)
table = widgets.TableView()
table.set_model(model)
# apply proxy to every 2nd column
# table.proxifier[:, ::2].highlight_current(mode="column")
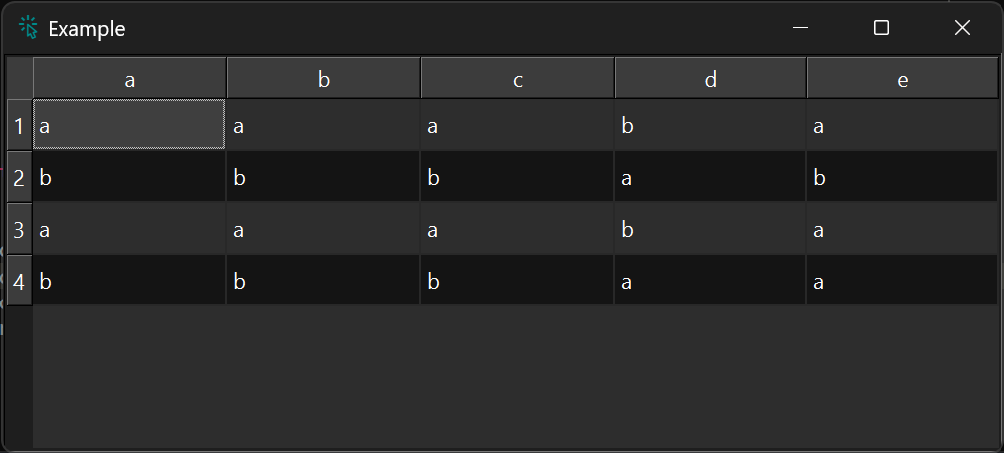
dct = dict(
a=["a", "b", "a", "b"],
b=["a", "b", "a", "b"],
c=["a", "b", "a", "b"],
d=["b", "a", "b", "a"],
e=["a", "b", "a", "a"],
)
model = gui.StandardItemModel.from_dict(dct)
table = widgets.TableView()
table.set_model(model)
# apply proxy to every 2nd column
table.proxifier[:, ::2].highlight_current(mode="row")
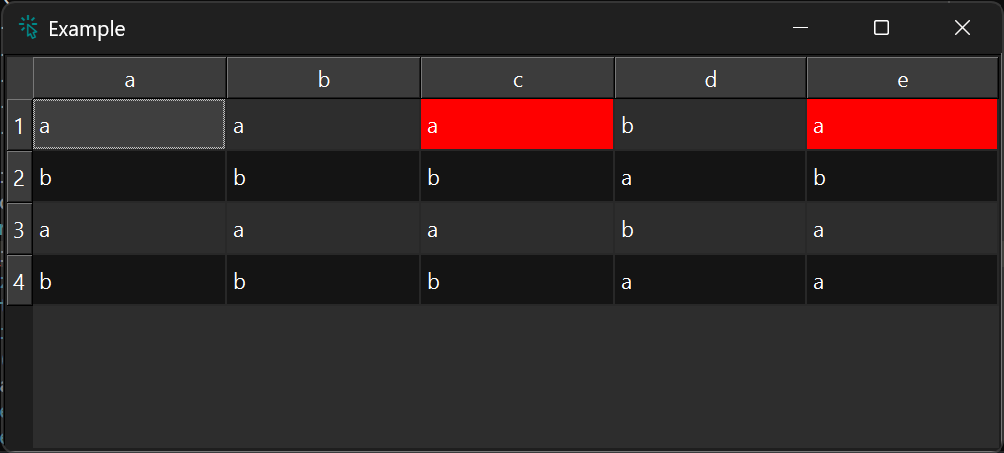
dct = dict(
a=["a", "b", "a", "b"],
b=["a", "b", "a", "b"],
c=["a", "b", "a", "b"],
d=["b", "a", "b", "a"],
e=["a", "b", "a", "a"],
)
model = gui.StandardItemModel.from_dict(dct)
table = widgets.TableView()
table.set_model(model)
# apply proxy to every 2nd column
table.proxifier[:, ::2].highlight_current(mode="column")
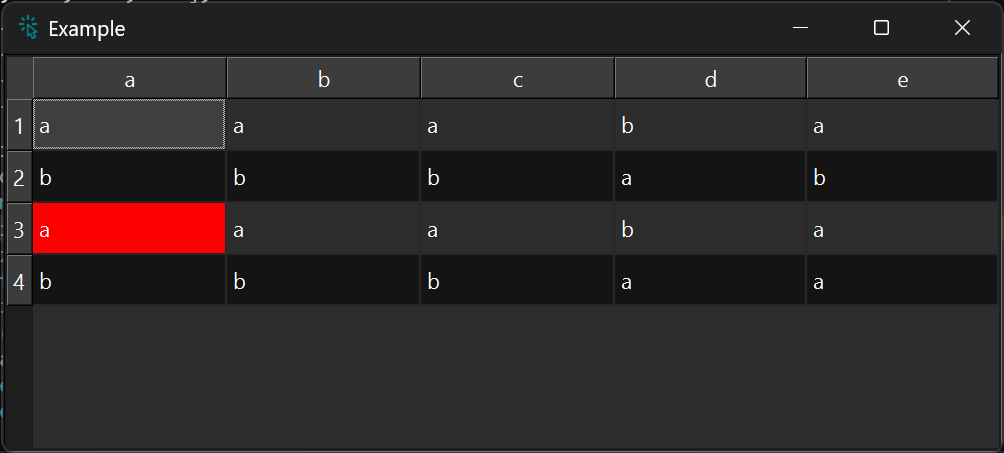
dct = dict(
a=["a", "b", "a", "b"],
b=["a", "b", "a", "b"],
c=["a", "b", "a", "b"],
d=["b", "a", "b", "a"],
e=["a", "b", "a", "a"],
)
model = gui.StandardItemModel.from_dict(dct)
table = widgets.TableView()
table.set_model(model)
# apply proxy to every 2nd column
table.proxifier[:, ::2].highlight_current(mode="all")
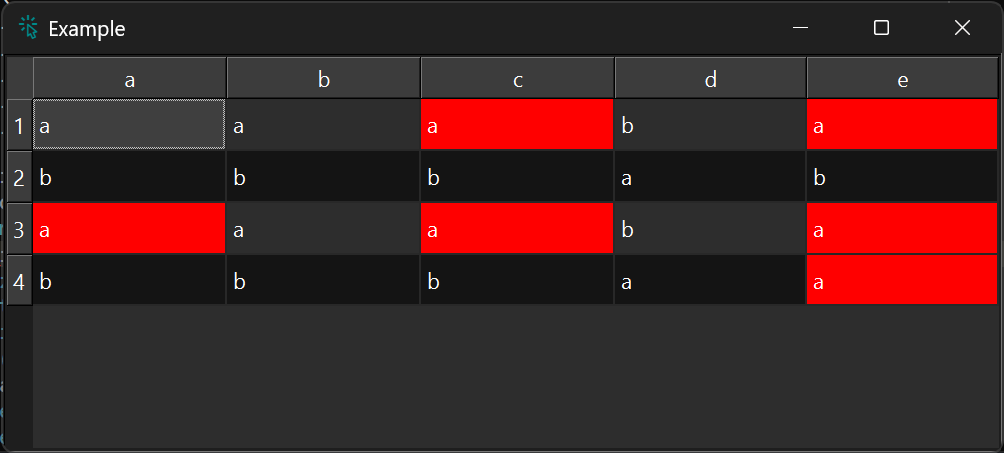
Example
model = MyModel()
table = widgets.TableView()
table.set_model(model)
table[:, :3].proxify.highlight_current(mode="all")
table.show()
# or
indexer = (slice(None), slice(None, 3))
proxy = itemmodels.SliceFilterProxyModel(indexer=indexer)
proxy.set_source_model(model)
table.set_model(proxy)
table.selectionModel().currentChanged.connect(proxy.highlight_index)
table.show()
Source code in prettyqt\itemmodels\proxies\slicehighlightcurrentproxymodel.py
13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 |
|
get_highlight_color() -> QtGui.QColor
get_highlight_mode() -> HighlightModeStr
get_highlight_role() -> constants.ItemDataRole
set_highlight_color(color: datatypes.ColorType)
set_highlight_mode(mode: HighlightModeStr)
Info
This is a slice proxy and can be selectively applied to a model. Read more about slices.
⌗ Property table
Qt Property | Type | Doc |
---|---|---|
objectName |
QString | |
sourceModel |
QAbstractItemModel | |
column_slice |
QVariantList | Column slice to include for the proxy |
row_slice |
QVariantList | Row slice to include for the proxy |
highlightMode |
QString | Highlight mode |
highlightColor |
QColor | Color to use for highlighting |
highlightRole |
PySide::PyObjectWrapper | ItemRole to use for highlighting |
highlight_column |
int | Currently highlighted column |
highlight_row |
int | Currently highlighted row |